Which UI do you use?
Pre built UI
Custom UI
How to enable debug logs
#
Enable backend logsimportant
This is only available on versions:
- supertokens-node >= v9.1.2
- supertokens-golang >= v0.5.5
- supertokens-python >= v0.6.3
Our backend SDK provides useful logs that can help with debugging. To enable logging, you need to set the debug setting to true
in the init
function call:
- NodeJS
- GoLang
- Python
- Other Frameworks
Important
For other backend frameworks, you can follow our guide on how to spin up a separate server configured with the SuperTokens backend SDK to authenticate requests and issue session tokens.
import supertokens from "supertokens-node";
supertokens.init({
debug: true,
supertokens: {
connectionURI: "...",
},
appInfo: {
apiDomain: "...",
appName: "...",
websiteDomain: "..."
},
recipeList: [/*...*/]
});
import "github.com/supertokens/supertokens-golang/supertokens"
func main() {
supertokens.Init(supertokens.TypeInput{
Debug: true,
Supertokens: &supertokens.ConnectionInfo{
ConnectionURI: "...",
APIKey: "...",
},
})
}
from supertokens_python import init, InputAppInfo, SupertokensConfig
init(
debug=True,
app_info=InputAppInfo(api_domain="...", app_name="...", website_domain="..."),
supertokens_config=SupertokensConfig(
connection_uri='...',
api_key='...'
),
framework='flask',
recipe_list=[
#...
]
)
Logs on your terminal have the following shape:
com.supertokens {"t": "2022-04-09T08:44:49.057Z", "sdkVer": "...", "message": "Started SuperTokens with debug logging (supertokens.init called)", "file": "..."}
t
: The time the log was generatedsdkVer
: Version of the SDK you are usingmessage
: The log messagefile
: The file and line number from where this log was generated.
#
Enable frontend logsAdd enableDebugLogs
when calling the init
function:
- ReactJS
- Angular
- Vue
Important
SuperTokens does not provide non-React UI components. So we will be using the
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import React from 'react';
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
enableDebugLogs: true,
appInfo: { /*...*/ },
recipeList: [/*...*/]
});
Important
SuperTokens does not provide non-React UI components. So we will be using the
supertokens-auth-react
SDK and will inject the React components to show the UI. Therefore, the code snippet below refers to the supertokens-auth-react
SDK.import React from 'react';
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
enableDebugLogs: true,
appInfo: { /*...*/ },
recipeList: [/*...*/]
});
import React from 'react';
import SuperTokens from "supertokens-auth-react";
SuperTokens.init({
enableDebugLogs: true,
appInfo: { /*...*/ },
recipeList: [/*...*/]
});
The above will print out supertokens debug log on the browser console:
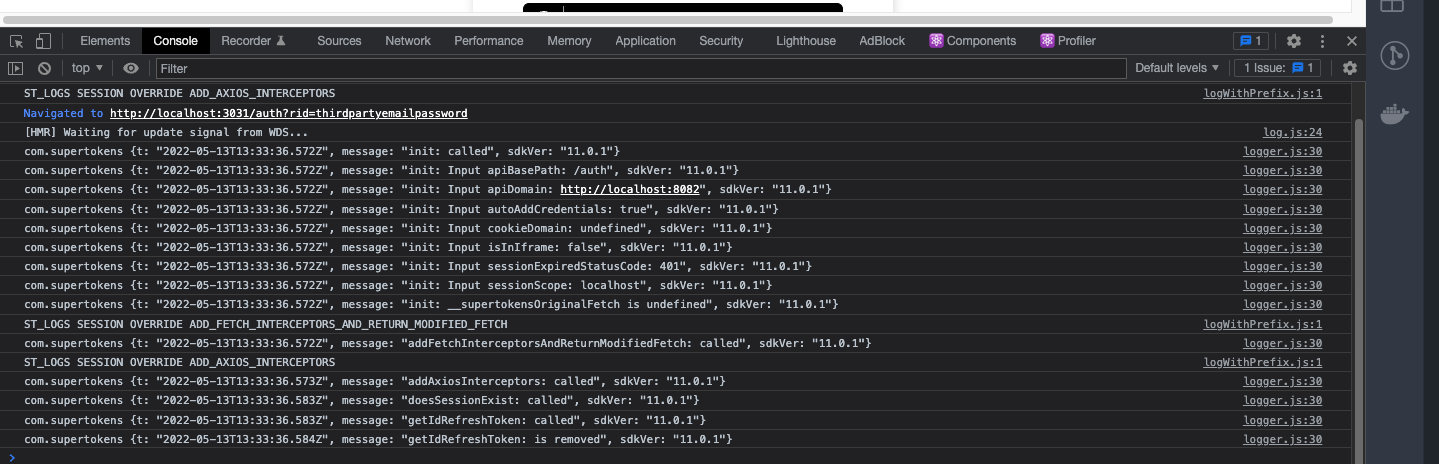